Tipos de eventos TextBox Excel VBA
Tipos de eventos TextBox Excel VBA são as formatação para exibir os dados via comando no Excel VBA. Vamos conhecer alguns comando e eventos mais utilizado na programação VBA.
Por padrão uma TextBox não tem nenhuma formatação e os comandos abaixo vai ajudar o seu projeto VBA ficar mais profissional.
Segue abaixo um formulário para cadastro de clientes onde foi colocado o evento Change para formatar no modelo CPF não permitindo erro de digitação.
Vamos aprender a formatar o campo CPF.
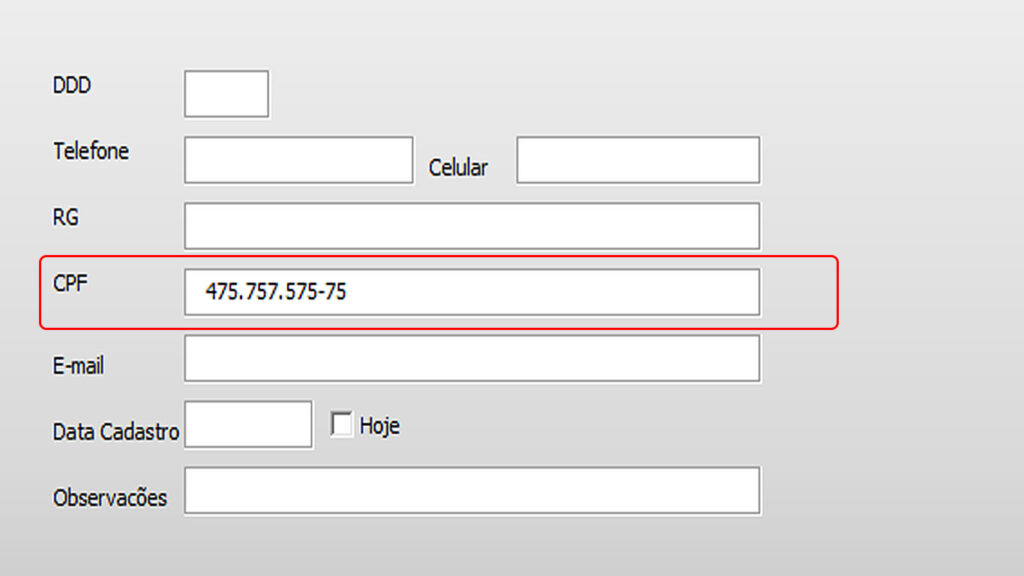
Utilizamos o comando abaixo para formatar a TextBox “txt_cpf” no evento KeyPress. Colocamos dentro da função também o comando permitindo somente digitação de números na TextBox.
Private Sub txt_cpf_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
txt_cpf.MaxLength = 14
Select Case KeyAscii
Case 8, 48 To 57 ‘ BackSpace e numericos
If Len(txt_cpf) = 3 Or Len(txt_cpf) = 7 Then
txt_cpf.Text = txt_cpf.Text & “.”
‘ SendKeys “{End}”, True
End If
If Len(txt_cpf) = 11 Then
txt_cpf.Text = txt_cpf.Text & “-“
‘ SendKeys “{End}”, True
End If
Case Else ‘ o resto é travado
KeyAscii = 0
End Select
End Sub
Vamos colocar também um código em nossa TextBox “txt_cpf” no evento KeyDown para não permitir o control + v no campo “txt_cpf”.
Private Sub txt_cpf_KeyDown(ByVal KeyCode As MSForms.ReturnInteger, ByVal Shift As Integer)
‘Proibe o control + v no campo senha
If (Shift = 2 And KeyCode = vbKeyV) Or (Shift = 1 And KeyCode = vbKeyInsert) Then
KeyCode = 0
End If
End Sub
Agora vamos aprender a formatar o campo data.
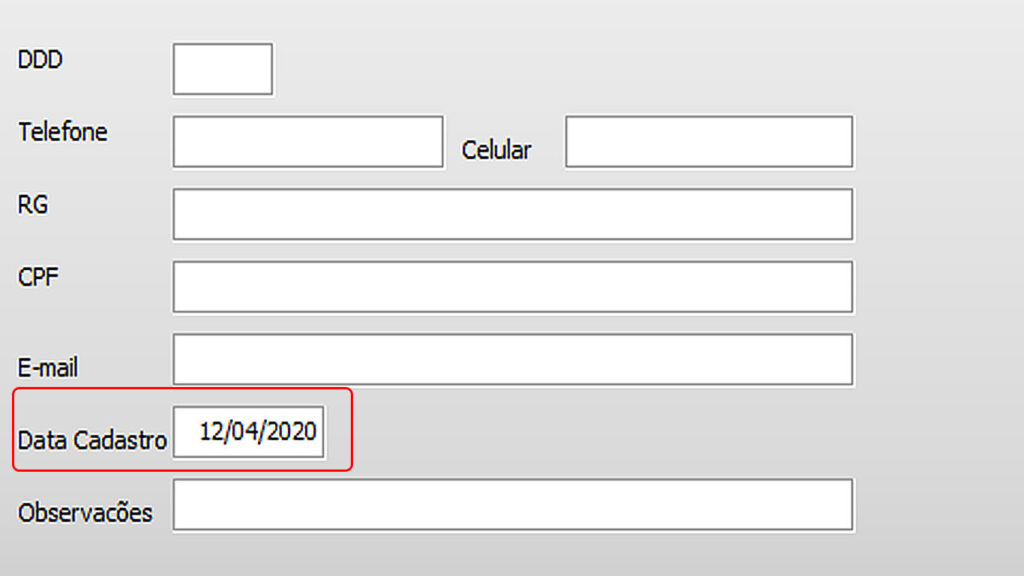
Utilizamos o comando abaixo para formatar a TextBox “txt_data” no evento KeyPress. Colocamos dentro da função também o comando permitindo somente digitação de números na TextBox.
Private Sub txt_data_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
Select Case KeyAscii
Case 8 ‘Aceita o BACK SPACE
Case 13: SendKeys “{TAB}” ‘Emula o TAB
Case 48 To 57
If txt_data.SelStart = 2 Then txt_data.SelText = “/”
If txt_data.SelStart = 5 Then txt_data.SelText = “/”
Case Else: KeyAscii = 0 ‘Ignora os outros caracteres
End Select
End Sub
Vamos aprender a formatar a campo nome.
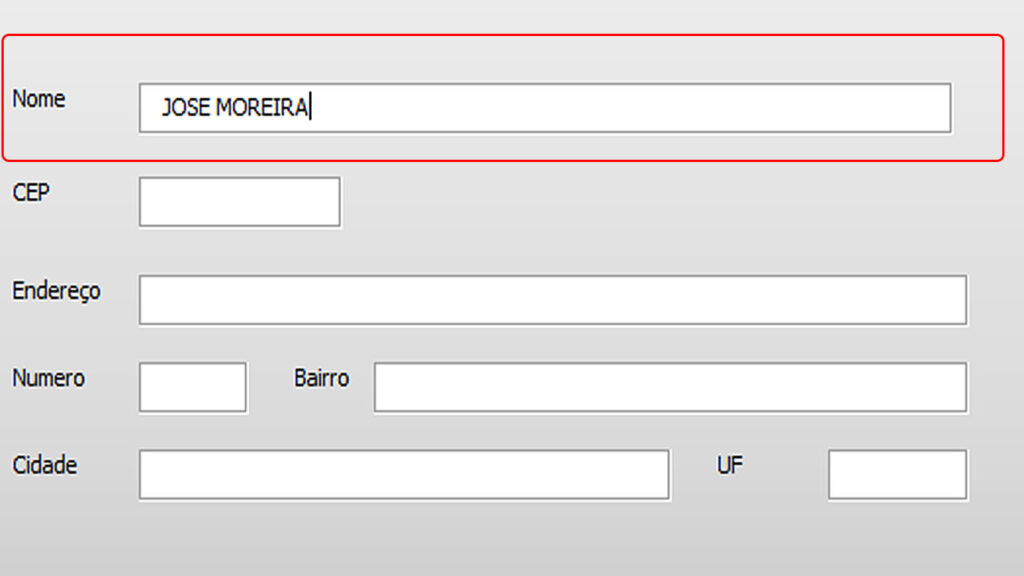
O comando utilizado abaixo vai permitir somente a digitação de letras maiúscula no TextBox “txt_nome” no evento KeyPress.
‘Aplicar formato Letra Maiúscula
KeyAscii = Asc(UCase(Chr(KeyAscii)))
Agora vamos aprender a formatar o campo CNPJ
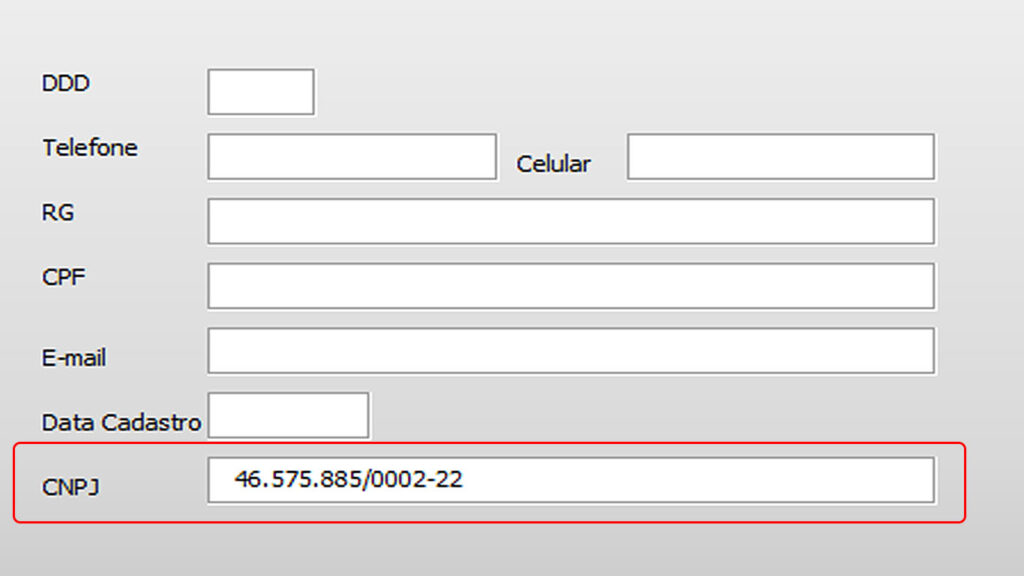
Comando utilizado para formatar tipo CNPJ no evento KeyPress da TextBox e colocamos também o comando permitindo somente digitação de números na TextBox.
Private Sub txt_cnpj_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
txt_cnpj.MaxLength = 18
Select Case KeyAscii
Case 8 ‘Aceita o BACK SPACE
Case 13: SendKeys “{TAB}” ‘Emula o TAB
Case 48 To 57
If txt_cnpj.SelStart = 2 Then txt_cnpj.SelText = “.”
If txt_cnpj.SelStart = 6 Then txt_cnpj.SelText = “.”
If txt_cnpj.SelStart = 10 Then txt_cnpj.SelText = “/”
If txt_cnpj.SelStart = 15 Then txt_cnpj.SelText = “-“
Case Else: KeyAscii = 0 ‘Ignora os outros caracteres
End Select
End Sub
Vamos aprender a formatar a campo CEP.
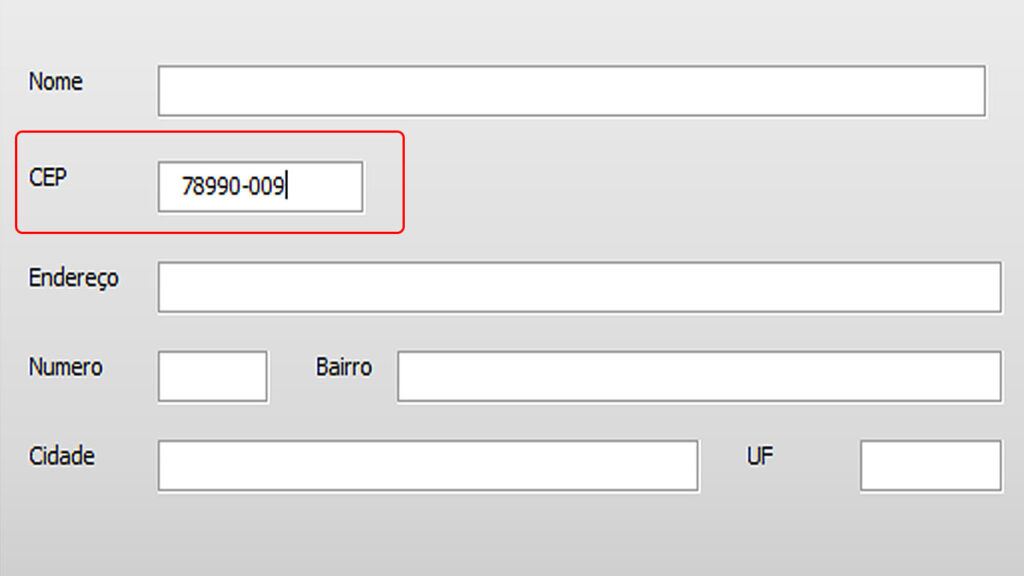
Comando utilizado para formatar tipo CEP no evento KeyPress da TextBox e colocamos também o comando permitindo somente digitação de números na TextBox.
Private Sub txt_cep_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
txt_cep.MaxLength = 9
Select Case KeyAscii
Case 8, 48 To 57 ‘ BackSpace e numericos
If Len(txt_cep) = 5 Then
txt_cep.Text = txt_cep.Text & “-“
End If
Case Else ‘ o resto é travado
KeyAscii = 0
End Select
End Sub
Agora vamos aprender a formatar o campo celular
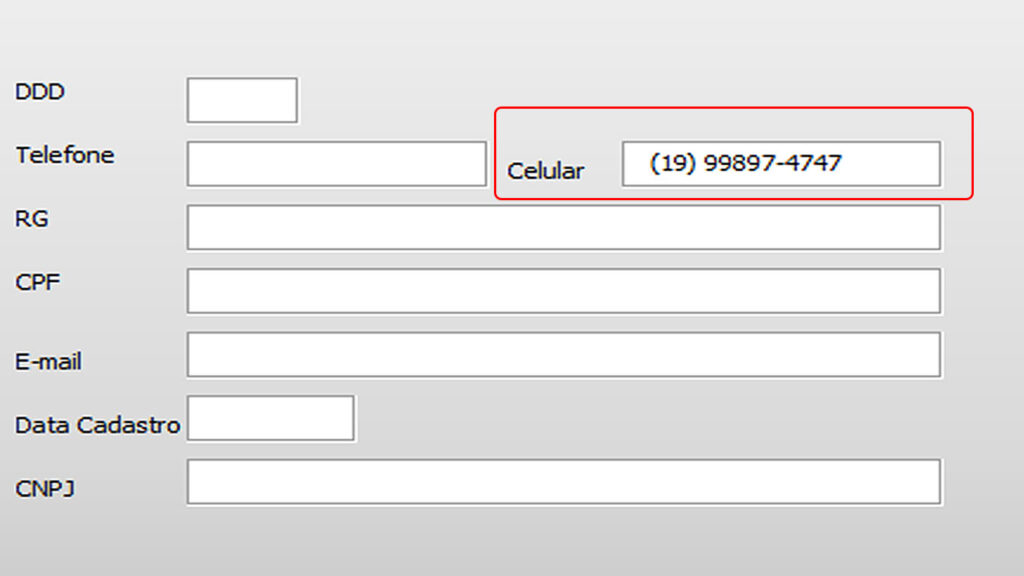
Comando utilizado para formatar tipo celular no evento KeyPress da TextBox e colocamos também o comando permitindo somente digitação de números na TextBox.
Private Sub txt_telefone_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
Select Case KeyAscii
‘ BackSpace e numericos
Case 8, 48 To 57
‘ Traço, só permite uma, para separador de decimais
Case 45
If InStr(1, txt_telefone.Text, Chr(45), vbTextCompare) > 1 Then _
KeyAscii = 0
Case Else ‘ o resto é travado
KeyAscii = 0
End Select
‘Limita a Qde de caracteres
txt_telefone.MaxLength = 15
‘Formato (xx) xxxx-xxxx
If Len(txt_telefone) = 0 Then
txt_telefone.Text = “(“
End If
If Len(txt_telefone) = 3 Then
txt_telefone.Text = txt_telefone & “) “
End If
If Len(txt_telefone) = 10 Then
txt_telefone.Text = txt_telefone & “-“
End If
End Sub
Vamos aprender a formatar a campo telefone fixo.
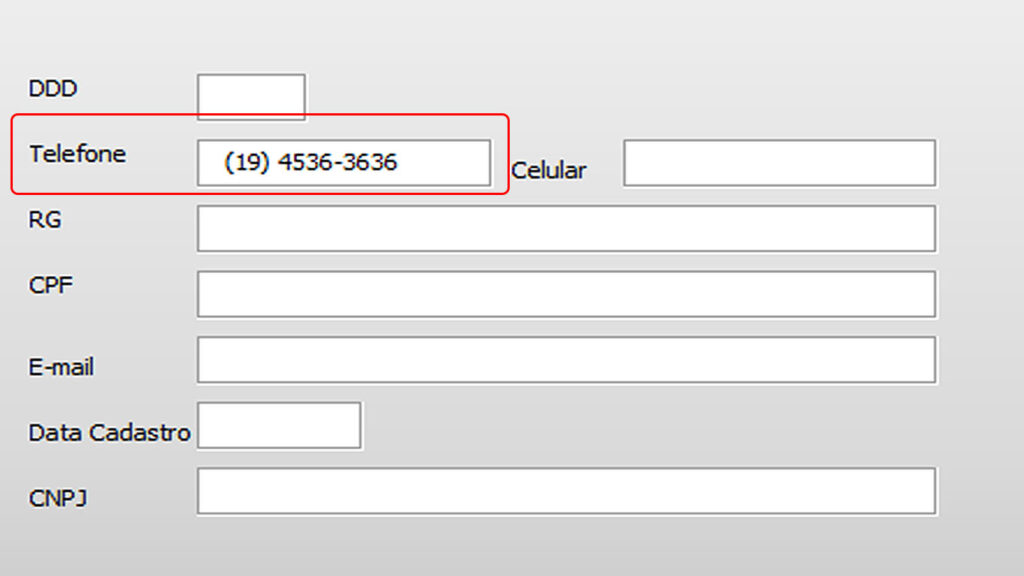
Comando utilizado para formatar tipo telefone fixo no evento KeyPress da TextBox e colocamos também o comando permitindo somente digitação de números na TextBox.
Private Sub txt_telefonefixo_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
Select Case KeyAscii
‘ BackSpace e numericos
Case 8, 48 To 57
‘ Traço, só permite uma, para separador de decimais
Case 45
If InStr(1, txt_telefonefixo.Text, Chr(45), vbTextCompare) > 1 Then _
KeyAscii = 0
Case Else ‘ o resto é travado
KeyAscii = 0
End Select
‘Limita a Qde de caracteres
txt_telefonefixo.MaxLength = 14
‘Formato (xx) xxxx-xxxx
If Len(txt_telefonefixo) = 0 Then
txt_telefonefixo.Text = “(“
End If
If Len(txt_telefonefixo) = 3 Then
txt_telefonefixo.Text = txt_telefonefixo & “) “
End If
If Len(txt_telefonefixo) = 9 Then
txt_telefonefixo.Text = txt_telefonefixo & “-“
End If
End Sub

Agora vamos aprender a formatar o campo RG
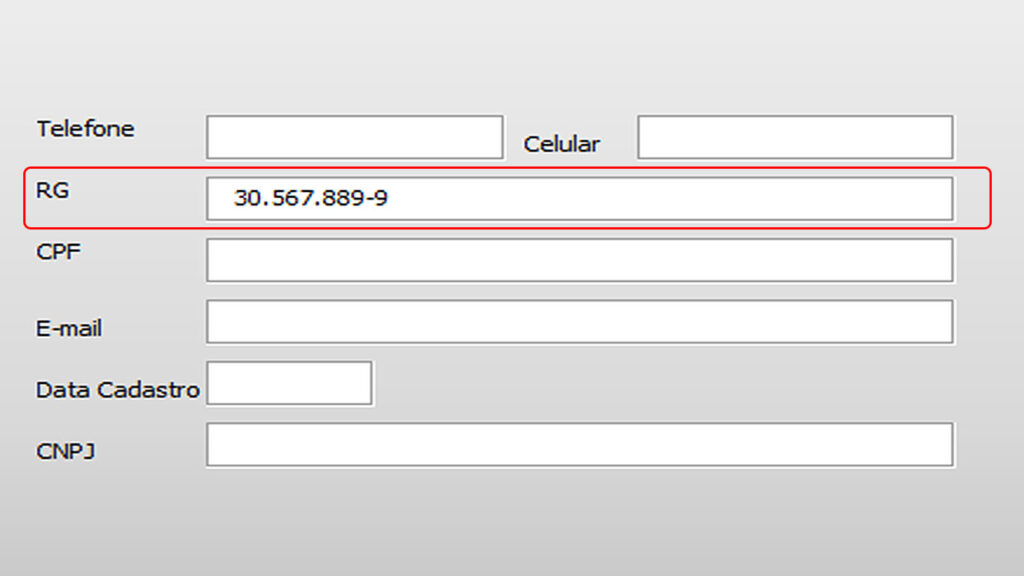
Comando utilizado para formatar tipo RG no evento KeyPress da TextBox e colocamos também o comando permitindo somente digitação de números na TextBox.
Private Sub txt_rg_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
txt_rg.MaxLength = 13
Select Case KeyAscii
Case 8, 48 To 57 ‘ BackSpace e numericos
If Len(txt_rg) = 2 Or Len(txt_rg) = 6 Then
txt_rg.Text = txt_rg.Text & “.”
‘ SendKeys “{End}”, True
End If
If Len(txt_rg) = 10 Then
txt_rg.Text = txt_rg.Text & “-“
‘ SendKeys “{End}”, True
End If
Case Else ‘ o resto é travado
KeyAscii = 0
End Select
End Sub
Tipos de eventos TextBox Excel VBA. Baixe a planilha usada neste artigo.
Olá, amigos! Inscreva-se em nosso canal do Youtube para não perder os próximos vídeos.
não consigo baixar
Olá amigo o navegador google chrome está com falhas para download favor usar outro navegador para baixar as planilhas.
Ola, e para moeda no modo keypress de uma texgtbox do form ou activex no vba.